Python 以其简单易懂的语法格式与其它语言形成鲜明对比,初学者遇到最多的问题就是不按照 Python 的规则来写,即便是有编程经验的程序员,也容易按照固有的思维和语法格式来写 Python 代码,有一个外国小伙总结了一些大家常犯的错误,16 Common Python Runtime Errors Beginners Find,我把他翻译过来并在原来的基础补充了我的一些理解,希望可以让你避开这些坑。
0、忘记写冒号
在 if、elif、else、for、while、class、def 语句后面忘记添加 “:”
if spam == 42
print('Hello!')
导致:SyntaxError: invalid syntax
1、误用 “=” 做等值比较
“=” 是赋值操作,而判断两个值是否相等是 “==”
if spam = 42:
print('Hello!')
导致:SyntaxError: invalid syntax
2、使用错误的缩进
Python用缩进区分代码块,常见的错误用法:
print('Hello!')
print('Howdy!')
导致:IndentationError: unexpected indent。同一个代码块中的每行代码都必须保持一致的缩进量
if spam == 42:
print('Hello!')
print('Howdy!')
导致:IndentationError: unindent does not match any outer indentation level。代码块结束之后缩进恢复到原来的位置
if spam == 42:
print('Hello!')
导致:IndentationError: expected an indented block,“:” 后面要使用缩进
3、变量没有定义
if spam == 42:
print('Hello!')
导致:NameError: name 'spam' is not defined
4、获取列表元素索引位置忘记调用 len 方法
通过索引位置获取元素的时候,忘记使用 len 函数获取列表的长度。
spam = ['cat', 'dog', 'mouse']
for i in range(spam):
print(spam[i])
导致:TypeError: range() integer end argument expected, got list. 正确的做法是:
spam = ['cat', 'dog', 'mouse']
for i in range(len(spam)):
print(spam[i])
当然,更 Pythonic 的写法是用 enumerate
spam = ['cat', 'dog', 'mouse']
for i, item in enumerate(spam):
print(i, item)
5、修改字符串
字符串一个序列对象,支持用索引获取元素,但它和列表对象不同,字符串是不可变对象,不支持修改。
spam = 'I have a pet cat.'
spam[13] = 'r'
print(spam)
导致:TypeError: 'str' object does not support item assignment 正确地做法应该是:
spam = 'I have a pet cat.'
spam = spam[:13] + 'r' + spam[14:]
print(spam)
6、字符串与非字符串连接
num_eggs = 12
print('I have ' + num_eggs + ' eggs.')
导致:TypeError: cannot concatenate 'str' and 'int' objects
字符串与非字符串连接时,必须把非字符串对象强制转换为字符串类型
num_eggs = 12
print('I have ' + str(num_eggs) + ' eggs.')
或者使用字符串的格式化形式
num_eggs = 12
print('I have %s eggs.' % (num_eggs))
7、使用错误的索引位置
spam = ['cat', 'dog', 'mouse']
print(spam[3])
导致:IndexError: list index out of range
列表对象的索引是从0开始的,第3个元素应该是使用 spam[2] 访问
8、字典中使用不存在的键
spam = {'cat': 'Zophie', 'dog': 'Basil', 'mouse': 'Whiskers'}
print('The name of my pet zebra is ' + spam['zebra'])
在字典对象中访问 key 可以使用 []
,但是如果该 key 不存在,就会导致:KeyError: 'zebra'
正确的方式应该使用 get 方法
spam = {'cat': 'Zophie', 'dog': 'Basil', 'mouse': 'Whiskers'}
print('The name of my pet zebra is ' + spam.get('zebra'))
key 不存在时,get 默认返回 None
9、用关键字做变量名
class = 'algebra'
导致:SyntaxError: invalid syntax
在 Python 中不允许使用关键字作为变量名。Python3 一共有33个关键字。
>>> import keyword
>>> print(keyword.kwlist)
['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
10、函数中局部变量赋值前被使用
someVar = 42
def myFunction():
print(someVar)
someVar = 100
myFunction()
导致:UnboundLocalError: local variable 'someVar' referenced before assignment
当函数中有一个与全局作用域中同名的变量时,它会按照 LEGB 的顺序查找该变量,如果在函数内部的局部作用域中也定义了一个同名的变量,那么就不再到外部作用域查找了。因此,在 myFunction 函数中 someVar 被定义了,所以 print(someVar) 就不再外面查找了,但是 print 的时候该变量还没赋值,所以出现了 UnboundLocalError
11、使用自增 “++” 自减 “--”
spam = 0
spam++
哈哈,Python 中没有自增自减操作符,如果你是从C、Java转过来的话,你可要注意了。你可以使用 “+=” 来替代 “++”
spam = 0
spam += 1
12、错误地调用类中的方法
class Foo:
def method1():
print('m1')
def method2(self):
print("m2")
a = Foo()
a.method1()
导致:TypeError: method1() takes 0 positional arguments but 1 was given
method1 是 Foo 类的一个成员方法,该方法不接受任何参数,调用 a.method1() 相当于调用 Foo.method1(a),但 method1 不接受任何参数,所以报错了。正确的调用方式应该是 Foo.method1()。
需要注意的是,以上代码都是基于 Python3 的,在 Python2 中即使是同样的代码出现的错误也不尽一样,尤其是最后一个例子。
关注公众号「Python之禅」,回复「1024」免费获取Python资源
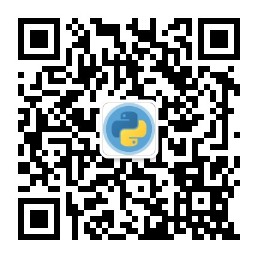